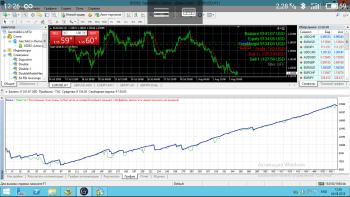
//+------------------------------------------------------------------+
//| Proba.mq4 |
//| Copyright 2018, AM2+axe44 |
//| http://www.forexsystems.biz |
//+------------------------------------------------------------------+
#property copyright "Copyright 2018, AM2+axe44 "
#property link "http://www.forexsystems.biz"
#property version "3.00"
//--- Inputs
extern double Lots = 3; // лот
extern double KLot = 1.5; // увеличение лота
extern int StopLoss = 50; // лось
extern int TakeProfit = 280; // язь
extern int TrailingStop = 20; // трал
extern int StartHour = 7; // час начала торговли
extern int Slip = 3; // реквот
extern int Count = 5; // баров для просчета
extern int Delta = 10; // расстояние от экстремума
extern int Expir = 10; // время жизни ордеров в минутах
extern int Spred = 4; // спред
extern int Counts = 5; // число поз
extern int Magic = 123; // магик
datetime t=1;int i;
// https://www.mql5.com/ru/signals/383802
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
//---
if(Digits==3 || Digits==5)
{
TakeProfit*=10;
StopLoss*=10;
Delta*=10;
Slip*=10;
Spred*=10;
}
//---
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Expert deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
Comment("");
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void PutOrder(int type,double price)
{
int r=0;
color clr=Green;
double sl=0,tp=0;
if(type==1 || type==3 || type==5)
{
clr=Red;
if(StopLoss>0) sl=NormalizeDouble(price+StopLoss*Point,Digits);
if(TakeProfit>0) tp=NormalizeDouble(price-TakeProfit*Point,Digits);
}
if(type==0 || type==2 || type==4)
{
clr=Blue;
if(StopLoss>0) sl=NormalizeDouble(price-StopLoss*Point,Digits);
if(TakeProfit>0) tp=NormalizeDouble(price+TakeProfit*Point,Digits);
}
r=OrderSend(NULL,type,Lots,NormalizeDouble(price,Digits),Slip,sl,tp,"",Magic,TimeCurrent()+Expir*60,clr);
return;
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void Trailing()
{
bool mod;
for(i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==Magic)
{
if(OrderType()==OP_BUY)
{
if(Bid-OrderOpenPrice()>TrailingStop*Point)
{
if(OrderStopLoss()<Bid-TrailingStop*Point)
{
mod=OrderModify(OrderTicket(),OrderOpenPrice(),Bid-TrailingStop*Point,OrderTakeProfit(),0,Yellow);
return;
}
}
}
if(OrderType()==OP_SELL)
{
if((OrderOpenPrice()-Ask)>TrailingStop*Point)
{
if((OrderStopLoss()>(Ask+TrailingStop*Point)) || (OrderStopLoss()==0))
{
mod=OrderModify(OrderTicket(),OrderOpenPrice(),Ask+TrailingStop*Point,OrderTakeProfit(),0,Yellow);
return;
}
}
}
}
}
}
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
int CountTrades()
{
int count=0;
for(i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==Magic)
{
if(OrderType()<2) count++;
}
}
}
return(count);
}
//+------------------------------------------------------------------+
//| Подсчет ордеров по типу |
//+------------------------------------------------------------------+
int CountOrders(int type)
{
int count=0;
for(i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==Magic)
{
if(OrderType()==type) count++;
}
}
}
return(count);
}
//+------------------------------------------------------------------+
//| Удаление отложенных ордеров |
//+------------------------------------------------------------------+
/*void DelOrder()
{
bool del;
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==Magic)
{
if(OrderType()>1) del=OrderDelete(OrderTicket());
}
}
}
}*/
//+------------------------------------------------------------------+
//| Expert tick function |
//+------------------------------------------------------------------+
void OnTick()
{
double up=High[iHighest(NULL,0,MODE_HIGH,Count,1)];
double dn=Low[iLowest(NULL,0,MODE_LOW,Count,1)];
if(TrailingStop>0) Trailing();
if((Ask-Bid)<Spred*Point)
{
if(CountOrders(2)<1 && Bid>up)
{
for(i=0;i<Counts;i++)
{
PutOrder(2,Bid-Delta*_Point-i*Delta*_Point*KLot);
}
}
if(CountOrders(3)<1 && Ask<dn)
{
for(i=0;i<Counts;i++)
{
PutOrder(3,Ask+Delta*_Point+i*Delta*_Point*KLot);
}
}
}
// if(CountTrades()>0) DelOrder();
}
//+------------------------------------------------------------------+
if(CountTrades()>0 && FindOrderType()==1 && (Bid-FindLastSellPrice())/Point>=Step)
{
// PutOrder(0,Ask);
PutOrder(1,Bid);
ModifyOrders(0);
ModifyOrders(1);
}
if(CountTrades()>0 && FindOrderType()==0 && (FindLastBuyPrice()-Ask)/Point>=Step)
{
// PutOrder(1,Bid);
PutOrder(0,Ask);
ModifyOrders(1);
ModifyOrders(0);
}
void ModifyOrders(int type)
{
double all=0,count=0,sl=0,tp=0;
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==Magic)
{
if(OrderType()==type)
{
all+=OrderOpenPrice();
count++;
}
}
}
}
//+------------------------------------------------------------------+
//| лимитник.mq4 |
//| Copyright 2018, axe44 |
//| http://axe44.opentraders.ru/bio/ |
//+------------------------------------------------------------------+
#property copyright "Copyright 2018, axe44"
#property link "http://axe44.opentraders.ru/bio/"
#property version "4.00"
#property strict
//--- Inputs
extern double Lots = 0.1; // лот
extern int Times = 30; // время жизни ордеров
extern int StopLoss = 30; // лось
extern int TakeProfit = 70; // язь
//extern bool Tr = 0; // Обычный трал да/нет
extern int MA = 40; // длинна МА
extern bool Stop = 0; // Стоп ордера
extern bool Limit = 1; // Лимит ордера
extern int Spred = 4; // спред
extern int steps = 50; // отступ
extern int Slip = 30; // реквот
extern int Magic = 123; // магик
datetime t=1;double xx,yy,zz;
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
int OnInit() //так приблизительно должно выглядеть
{
//---
if(Digits==3 || Digits==5)
{
TakeProfit*=10;
StopLoss*=10;
steps*=10;
Slip*=10;
Spred*=10;
// NoLoss1*=10;
// MinProfit*=10;
}
//---
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Expert deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
Comment("");
}
//+------------------------------------------------------------------+
//Берём уровень текущую цену за основу, и пусть это будет икс. Берём скользящую среднию цену за игрек.
//Так же нам нужны такие величины как время в днях, шаг.
//Если икс больше/меньше скользящей средней на шаг, то выставляем стоп ордер, прописав время его жизни
//TimeCurrent()+60*60*60*24* время в днях
//в днях, прибыль и лося. Выставляем ордер через час/четыре часа/день по усмотрению. |
//+------------------------------------------------------------------+
void PutOrder(int type,double price)
{
int r=0;
color clr=Green;
double sl=0,tp=0;
if(type==5||type==3)
{
clr=Red;
if(steps>0) sl=NormalizeDouble(price+StopLoss*Point,Digits);
if(steps>0) tp=NormalizeDouble(price-TakeProfit*Point,Digits);
r=OrderSend(NULL,type,Lots,price,Slip,sl,tp,"",Magic,TimeCurrent()+Times*60*60,clr);// время истечения TimeCurrent()+Times*60*60
}
if(type==2||type==4)
{
clr=Blue;
if(steps>0) sl=NormalizeDouble(price-StopLoss*Point,Digits);
if(steps>0) tp=NormalizeDouble(price+TakeProfit*Point,Digits);
r=OrderSend(NULL,type,Lots,price,Slip,sl,tp,"",Magic,TimeCurrent()+Times*60*60,clr);
}
return;
}
//+------------------------------------------------------------------+
//| Expert tick function |
//+------------------------------------------------------------------+
void OnTick()
{
if(t!=Time[0]&&(Ask-Bid)<Spred*Point)
{
NormalizeDouble(xx=Bid,Digits);
NormalizeDouble(yy=iMA(NULL,0,MA,0,MODE_SMMA,PRICE_MEDIAN,0),Digits);
NormalizeDouble(zz=xx-yy,Digits);
if(xx<yy)NormalizeDouble(zz=yy-xx,Digits);
//Если икс больше/меньше скользящей средней на шаг, то выставляем стоп ордер и лимит, прописав время его жизни
Print("длинна отступа ",steps*Point,", разница равна ",zz);
if( AccountBalance()>300) if(zz>steps*Point)
{
if (Stop==1)
if (xx<yy){ PutOrder(4,NormalizeDouble(yy+StopLoss*Point,Digits));}
if (Limit==1)
if (xx<yy){ PutOrder(3,NormalizeDouble(yy,Digits));}
if (Stop==1)
if (xx>yy){ PutOrder(5,NormalizeDouble(yy-StopLoss*Point,Digits));}
if (Limit==1)
if (xx>yy){ PutOrder(2,NormalizeDouble(yy,Digits));}
}
t=Time[0];
}
}
Код не работает.
Вдруг зациклился сам на себе.
axe44